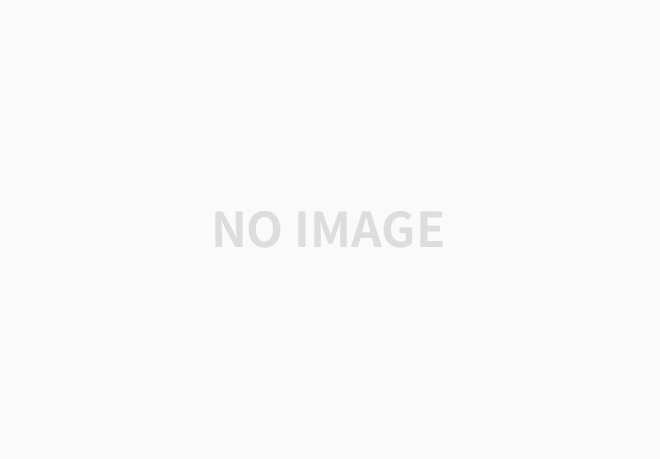
Check 01 - schema.prisma(prisma schema) 와 *.typeDefs.js(graphql schema) 의 sync를 맞춘다.
schema.prisma 파일
1
2
3
4
5
6
7
8
9
10
|
model User {
id Int @id @default(autoincrement())
firstName String
lastName String?
username String @unique
email String @unique
password String
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
|
cs |
users.typeDefs.js 파일
1
2
3
4
5
6
7
8
9
10
11
12
13
|
import { gql } from "apollo-server";
export default gql`
type User {
id: String!
firstName: String!
lastName: String
username: String!
email: String!
createdAt: String!
updatedAt: String!
}
`;
|
cs |
Check 02 - mutation resolver 를 만들때는 언제나 export default 를 사용한다.
1
2
3
4
5
|
export default {
Mutation: {
createAccount: (_, args) => {}
}
}
|
cs |
- createAccount 의 첫번째 인자 _ 는 root, 두번째 인자 args 는 사용자가 입력하는 데이터 이다.
Check 03 - prisma CRUD
https://www.prisma.io/docs/concepts/components/prisma-client/crud
CRUD (Reference)
How to perform CRUD with Prisma Client.
www.prisma.io
Check 04 - async & await
https://joshua1988.github.io/web-development/javascript/js-async-await/
자바스크립트 async와 await
(중급) 자바스크립트 개발자를 위한 async, await 사용법 설명. 쉽게 알아보는 자바스크립트 async await 개념, 사용법, 예제 코드, 예외 처리 방법
joshua1988.github.io
- async와 await는 자바스크립트의 비동기 처리 패턴 중 가장 최근에 나온 문법이다.
- async 함수 속에 있는 await 메서드는 순서를 보장받는다
Check 05 - bcrypt
- 설치법 : npm i bcrypt
- hash 는 단방향 function 이여서 암호화 할수는 있어도 복호화는 불가능하다.
사용법
1
2
3
|
import bcrypt from "bcrypt";
const uglyPassword = await bcrypt.hash(password, 10);
|
cs |
'Apollo GraphQL Prisma' 카테고리의 다른 글
GraphQL (0) | 2021.06.18 |
---|---|
인스타그램 클론코딩 BACKEND 만들기 #11 - Login (0) | 2021.06.17 |
인스타그램 클론코딩 BACKEND 만들기 #9 - Dotenv (0) | 2021.06.16 |
인스타그램 클론코딩 BACKEND 만들기 #8 - graphql-tools (0) | 2021.06.16 |
인스타그램 클론코딩 BACKEND 만들기 #7 - Prisma Client (0) | 2021.06.16 |